PHP MVC与数据库交互
分类:技术文档
时间:2024-07-04 22:13
浏览:128
概述
您可以创建用户管理应用程序,该应用程序使用 PHP MVC 与 MySQL 数据库交互。
内容
php mvc 架构模式可将交互式数据库的应用程序代码分为逻辑、界面和模型组件,实现维护和管理的便利。在实战案例中,mvc 用于构建用户管理应用程序,包括创建数据库、表、模型、视图和控制器,其中模型负责数据库交互,控制器验证数据并创建用户,视图则显示注册表单。
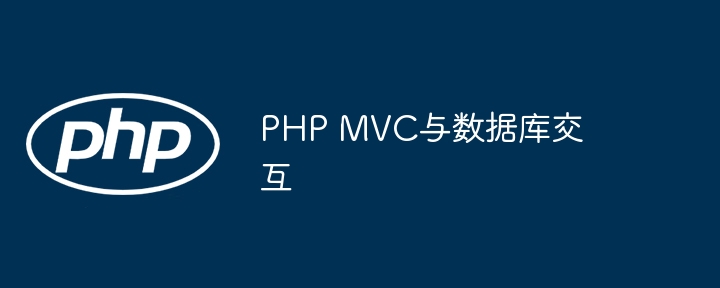
PHP MVC 与数据库交互:一个实战案例
Model-View-Controller (MVC) 架构模式是一种流行的软件设计方法,将应用程序逻辑、用户界面和数据模型分离为不同的组件。MVC 架构使代码更容易维护和管理,并且非常适合与数据库交互。
实战案例:创建用户管理应用程序
立即学习“PHP免费学习笔记(深入)”;
为了展示 PHP MVC 与数据库交互,我们创建一个简单的用户管理应用程序。此应用程序允许用户注册、登录和查看个人资料。
首先,为应用程序创建数据库:
1 | CREATE DATABASE user_management;
|
然后,使用以下模式创建 users 表:
1 2 3 4 5 6 7 | CREATE TABLE users (
id INT NOT NULL AUTO_INCREMENT,
username VARCHAR (255) NOT NULL ,
email VARCHAR (255) NOT NULL ,
password VARCHAR (255) NOT NULL ,
PRIMARY KEY (id)
);
|
接下来,设置 MVC 架构:
Model: UserModel.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | class UserModel
{
private $db ;
public function __construct()
{
$this ->db = new PDO( '<a style=' color:#f60; text-decoration:underline; ' href="https://www.php.cn/zt/15713.html" target="_blank">mysql</a>:host=localhost;dbname=user_management' , 'username' , 'password' );
}
public function createUser( $data )
{
$stmt = $this ->db->prepare( 'INSERT INTO users (username, email, password) VALUES (:username, :email, :password)' );
$stmt ->bindParam( ':username' , $data [ 'username' ]);
$stmt ->bindParam( ':email' , $data [ 'email' ]);
$stmt ->bindParam( ':password' , $data [ 'password' ]);
$stmt ->execute();
}
}
|
View: user_view.php
1 2 3 4 5 6 7 | <form action= "register.php" method= "POST" >
<input type= "text" name= "username" placeholder= "用户名" >
<input type= "email" name= "email" placeholder= "邮箱" >
<input type= "password" name= "password" placeholder= "密码" >
<button type= "submit" >注册</button>
</form>
|
Controller: UserController.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | class UserController
{
private $userModel ;
public function __construct()
{
$this ->userModel = new UserModel();
}
public function register()
{
if ( $_SERVER [ 'REQUEST_METHOD' ] == 'POST' ) {
$data = $_POST ;
if ( $this ->userModel->createUser( $data )) {
header( 'Location: login.php' );
exit ;
} else {
}
}
include 'user_view.php' ;
}
}
|
使用此代码,您可以创建用户管理应用程序,该应用程序使用 PHP MVC 与 MySQL 数据库交互。
评论